The fact that the MvcScaffolding package uses SQL Express by default can be both good and deceiving at the same time.
Using an Existing Database (not Code-First)
I used the package on a MVC3 project to scaffold the repository models and controllers for an existing SQL Server Standard database (not SQL Express) that had an existing Ado.Net Entity Framework model. The EF connection string obviously pointed to the existing database. However when running the application, the Index pages didn’t list any of the existing data from my database.
If this looks like the same problem you are having, feel free to skip to the bottom for the solution (convention over configuration).
Where’s the data?
I could add records, but they didn’t show up in the existing database. There was no Express database .mdf file in the application’s App_Data folder. There were no additional connection strings (other than the EF connection to the existing database) in the web.config files or any of the dbcontext class files. I profiled the existing database with SQL Profiler and the existing database was completely untouched. I even added a <remove name=”LPMEntities” /> line before the EF connection string to make sure any default connection from the server root or machine.config was taken out of the inheritance tree (similar to what you would do for the aspnetdb membership database if you are using a full SQL Server instance).
The new data was being stored somewhere, but where? In a newly created SQL Express database.
How do I See the Data?
Open your SQL Server Management Studio (SSMS) or the version of SSMS for SQL Express. In the Object Explorer window, click Connect. Use .\sqlexpress as the server to connect to using Windows Authentication and voila the new mysterious “hidden” database.
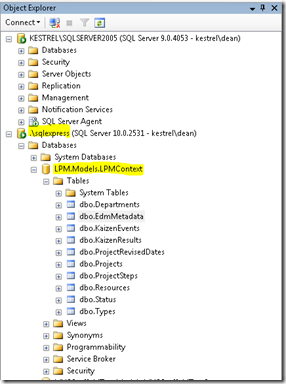
I hope this helps to clarify for other people attempting to use the MvcScaffoling in a Database-First scenario.
The Solution – Understand the Convention over Configuration
It took me a while to find a small subtle, yet crucial, detail in Scott Guthrie’s Using EF “Code First” with an Existing Database.
The following note is stated at the end of Step 5 – Configuring our Database Connection String:
EF “code first” uses a convention where context classes by default look for a connection-string that has the same name as the context class. Because our context class is called “Northwind” it by default looks for a “Northwind” connection-string to use. Above our Northwind connection-string is configured to use a local SQL Express database. You can alternatively point it at a remote SQL Server.
In my case the Entity Framework connection string in the web.config was named “LPMEntities”. The class implementing the DbContext is named LPMContext as shown below.
namespace LPM.Models
{
public class LPMContext : DbContext
{
public DbSet<LPM.Type> Types { get; set; }
additional stuff here...
}
}
Since LPMEntities is not the context class name, the EF generates a local SQL Express database.
I just added a connection string to the web.config with a name of LPMContext (same name as the context class) and my MVC forms are populated from the existing database.
Victory!
photo credit: Si1very / CC BY-SA 2.0